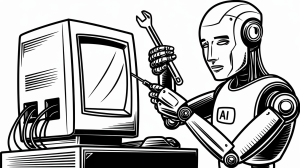
Function Calling: The Secret Sauce Behind Great Code
Introduction:
If you’ve ever been knee-deep in a project and wondered how software actually works, let me introduce you to the unsung hero: function calling. It’s the simple yet powerful mechanism that keeps our code clean, reusable, and (hopefully) bug-free. Sure, it’s not as glamorous as AI or blockchain, but trust me—without it, those fancy buzzwords wouldn’t exist.
What Is Function Calling, Really?
At its core, function calling is like texting your most reliable friend. You send them a message (the arguments), they do the task you asked (the function’s logic), and they get back to you with an answer (the return value). Easy, right?
Take this example:
def make_coffee(type, size):
return f”Here’s your {size} {type} coffee!”
When you call make_coffee(“latte”, “large”), the function works its magic and delivers:
“Here’s your large latte coffee!”
But behind the scenes, things get a little more complicated. Your computer is juggling memory, keeping track of where the function lives, and making sure it doesn’t accidentally serve you a cappuccino instead.
Then there’s the next-level stuff, like higher-order functions—functions that take other functions as arguments. If that sounds fancy, think of it as delegating your coffee order to a friend who knows your exact preferences:
def order_coffee(customizer):
return customizer(“espresso”, “medium”)
order_coffee(lambda type, size: f”A {size} {type} with oat milk, please!”)
# Returns: “A medium espresso with oat milk, please!”
Yes, your functions can be as fancy and customizable as your coffee order.
Why It Matters:
Here’s the thing—function calling isn’t just a “nice-to-have.” It’s what keeps our projects from descending into chaos. By breaking tasks into bite-sized chunks, it makes code more readable, testable, and shareable.
In team projects, functions act as contracts. You know exactly what a function expects and what it gives back, so there’s no need to dig through a hundred lines of someone else’s code (bless).
But beware: function calling can turn on you. Mismanage it, and you’ll end up with stack overflows (where your computer just gives up) or recursive nightmares. And if you’ve ever tried debugging a spaghetti mess of function calls at 2 AM, you know the pain is real.
How to Use Function Calling Like a Pro:
If you want to take your function game to the next level, here are some tips I’ve learned the hard way:
1. Understand the Call Stack: Picture a stack of plates—each function call adds a plate, and the stack can only handle so many before it collapses. (Don’t overload it.)
2. Keep Arguments Simple: Don’t hand a function a 20-page PDF if all it needs is the title. Pass just enough information to get the job done.
3. Use Asynchronous Calls: When your app needs to multitask—like fetching data from an API—learn to use async/await. Nobody likes waiting.
4. Write Reusable Functions: Think “one function, one job.” It’s like the single-tasking of the programming world, and it works.
Why It’s Kind of Amazing:
Here’s the part I love most: once you understand function calling, you start to see the beauty in it. It’s simple enough for small tasks but powerful enough to scale into something massive, like chaining middleware in a Node.js app or handling hundreds of API requests in parallel.
Honestly, function calling feels like having a superpower. It’s how we turn chaos into order, ideas into reality, and coffee orders into… well, actual coffee.
Conclusion:
So yeah, function calling might not be the flashiest thing in programming, but it’s the glue that holds everything together. Whether you’re just starting out or writing enterprise-level apps, understanding how to call, delegate, and manage functions is what separates good code from great code.
Now, if only debugging recursive calls were as easy as ordering coffee. But that’s a story for another day.